I’ve put in github my django program to monitor certificates – django-certiffy
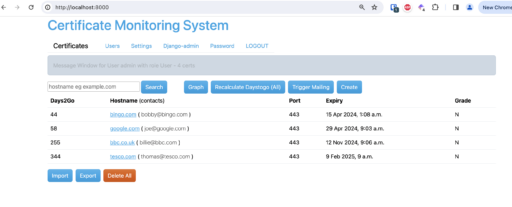
It can be deployed quite quickly and easily for testing:
git clone https://github.com/bradymd/django-certiffy.git
cd django-certiffy
python3 -m venv venv && source venv/bin/activate
cd django_certiffy_project
python3 -m pip install -r requirements.txt
python3 -m pip install --upgrade pip
rm db.sqlite3
python manage.py migrate --fake-initial
python manage.py migrate --run-syncdb
DJANGO_SUPERUSER_USERNAME=admin DJANGO_SUPERUSER_PASSWORD=psw python manage.py createsuperuser --email=admin@example.com --noinput
python manage.py runserver
I find it useful with nearly 300 certificates on our campus being tracked and replaced, its a daily task now. This helps generate keys and Certifcate Signing Requests, keep track of the owners, give people enough warning, and help see what is coming up. Oh, and it grades them using Qualys SSL Labs.