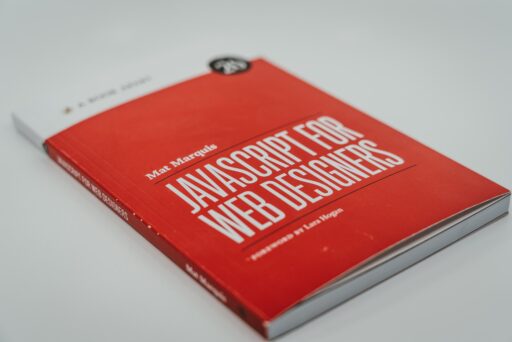
This is a nodejs program that provides access to a backend file. It’s acting like a simple API to pick out individual lines. e.g. http://hostname:3000/daystogo/ftp.example.com
It would pick out the line in the file with ftp.example.com in it. Simple.
onst express = require('express')
const app = express()
const fs = require("fs")
// have to read data each call as the file gets changed
function readdata() {
path = "/home/cert-api/results.csv"
var buffer = fs.readFileSync(path,{encoding:'utf8', flag:'r'})
var data = buffer.toString().split("\n")
return data
}
// This takes a parameter and picks it the line out of the file
app.get('/daystogo/:url',(req, res) => {
var output
var data=readdata()
output = ""
for(let row in data) {
if (data[row].match(req.params['url'])) {
output = output.concat(data[row])
}
}
res.end(output)
})
app.get('/', (req, res) => {
output=`<html>
<head><title>Certificate API</title></head>
<body>
<h1>Certificate API</h1><p>
<li>/daystogo-h - see the whole list in human readable form</li> \
<li>/daystogo-csv - the list in csv format</li>
<li>/daystogo/sample.example.com - a single entry in the file </li> </p>
</body>
</html> `
res.send(output)
})
app.get('/daystogo-h',(req, res) => {
var output
var data=readdata()
output=""
output = output.concat("<head><body>")
output = output.concat("<table><body>")
for (const line of data) {
row=line.split(",")
output=output + "<tr>"
for (item in row) {
output=output + "<td>" + row[item] + "</td>"
}
output=output + "</tr>"
}
output = output.concat("</table></body></html>")
res.send(output)
})
app.get('/daystogo-csv',(req, res) => {
var output
var data=readdata()
output = ""
for(row in data) {
output = output.concat(data[row])
output = output.concat("<br>")
}
res.send(output)
})
const server = app.listen(3000,'cert-api.example.com', () => {
console.log('Server ready')
})
process.on('SIGTERM', () => {
server.close(() => {
console.log('Process terminated')
})
})
To make it run as a daemon on linux we creat a file /lib/systemd/system/cert-api.service
[Unit]
Description=cert-api
After=network.target
[Service]
Type=simple
User=cert-api
ExecStart=/usr/bin/node /home/cert-api/cert-api.js
WorkingDirectory=/home/cert-api
Restart=on-failure
[Install]
WantedBy=multi-user.target
And then we make this a daemon:
systemctl daemon-reload
systemctl enable cert-api --now