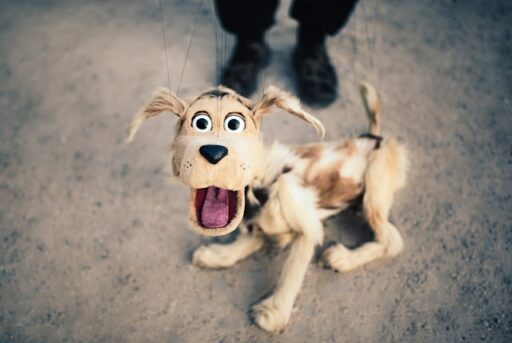
puppet ssl clean --localca
major="$(/opt/puppetlabs/bin/facter os.release.major)"
dnf remove puppet6-release puppet-agent -y
rpm -Uvh https://yum.puppet.com/puppet7-release-el-${major}.noarch.rpm
dnf install puppet-agent -y
/opt/puppetlabs/bin/puppet config set server puppet7master --section agent
hostname |grep la && sed -i=.bak -e '$a\192.168.1.149 vuh-lb-puppetmaster7' /etc/hosts
/opt/puppetlabs/bin/puppet config set environment common --section agent
/opt/puppetlabs/bin/puppet config set waitforcert 20s --section agent
/opt/puppetlabs/bin/puppet ssl bootstrap
echo run "puppetserver ca sign --all" on the master and then continue
read yn
puppet agent -t
puppet resource service puppet ensure=running enable=true
This method is a fresh start so removing the puppet6 certificates, as the puppetmaster is changing.