When you build a machine, getting the security settings right in your servers is very hard and so is keeping them up-to-date. There is nothing more useful than https://ssl-config.mozilla.org to help in this regard.
OK so a Python script you can run which will extract the useful code portion and doesn’t involve you cutting-and-pasting between your browser and your remote machine. It just pops out the code section. I typically use it to pull the list of SSLCiphers in apache or nginx.
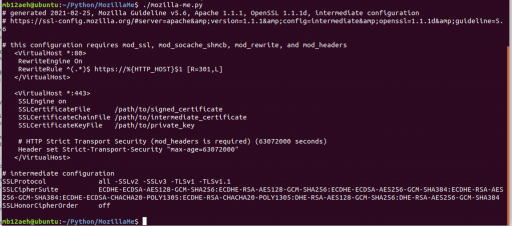
#!/usr/bin/env python3
#
# requirements.txt
# beautifulsoup4==4.9.3
# selenium==3.141.0
# soupsieve==2.2
# urllib3==1.26.3
# On centos:
# python3 -m venv MozillaMe
# cd MozillaMe
# source bin/activate
# pip3 list
# pip3 install selenium
# pip3 install beautifulsoup4
# Had to get the geckodriver from here, and put in /usr/local/bin
# https://github.com/mozilla/geckodriver/releases/tag/v0.29.0
# sudo yum install firefox -y
# On debian:
# apt install firefox-geckodriver
#
import ssl
import sys
import re
from selenium import webdriver
from bs4 import BeautifulSoup as bs
import ssl;
# The mozilla config site needs a URL like this - leave it here for reference
#url='https://ssl-config.mozilla.org/#server=nginx&version=1.17.7&config=intermediate&openssl=1.1.1d&guideline=5.6'
# OK this is because we have html < and > codes in the output
# To display I had to put a space between & lt; and & gt; which will need
# removing if you snip this code
def mung(text):
TAG_RE = re.compile(r'<[^>]+>')
txt=TAG_RE.sub('',str(text))
TAG_RE = re.compile(r'& lt;')
txt=TAG_RE.sub(' >',str(txt))
TAG_RE = re.compile(r'& gt;')
txt=TAG_RE.sub('>',str(txt))
return txt
# we can pull the version of openssl is on this running machine
version = ssl.OPENSSL_VERSION.split()[1]
# the server variable is typically nginx or apache but can be much more - see the website
server = "apache"
#server = "nginx"
url='https://ssl-config.mozilla.org/#server=' + server + '&version=' + version + '&config=intermediate&openssl=1.1.1d&guideline=5.6'
try:
fireFoxOptions = webdriver.FirefoxOptions()
fireFoxOptions.set_headless()
driver = webdriver.Firefox(firefox_options=fireFoxOptions)
driver.get(url)
myhtml = driver.page_source
htmlcontent= bs(myhtml, "html.parser")
codebit = htmlcontent.find("code", {"id": "output-config"})
code=mung(codebit)
print(code)
finally:
try:
driver.close()
except:
pass
sys.exit()